Table of Contents
Next.js 13 recap
Next.js has quickly gained popularity for building web applications. There has been a rise in adoption of Next.js among developers because of its ease of use and robust features. Vercel, made by the creators of Next.js, reported that there were over three million downloads per week in 2024. Well-known applications like Nike, Hulu, and Twitch have implemented Next.js for better performance and user experience.
Given the rapid pace of development and the ever-changing environment of online technologies, keeping up with the latest versions of frameworks like Next.js is essential. This ensures that developers can incorporate new features, optimizations, and upgrades to keep apps competitive and efficient.
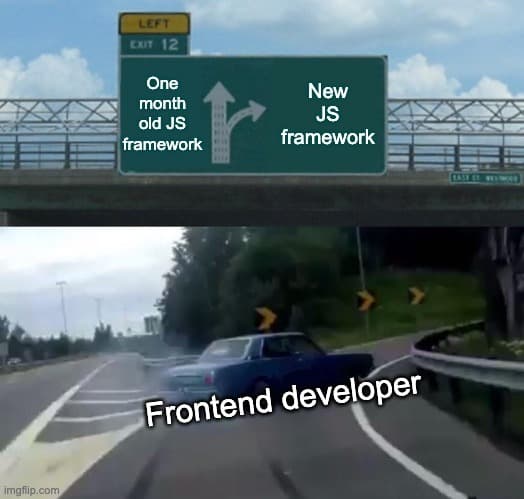
In this blog, we will look at the main differences and similarities between Next.js 13 and 14, and how you can select the best version for your application.
Next.js 13 recap
Next.js 13 introduced several improvements and new features over previous versions that enhanced the development experience and application performance. Key features included:
Improved Fast Refresh: Enabling developers to see changes instantly without losing component state.
Enhanced Image Optimization: Offering better default settings and improved support for modern image formats.
Built-in CSS and Sass Support: Allowing developers to seamlessly integrate styles without additional configuration.
If you would like a more in-depth review on Next.js 13, check out this article.
Similarities between Next.js 13 and 14
Despite the introduction of Next.js 14, several core features and benefits remain unchanged from Next.js 13:
Server-Side Rendering (SSR): Both versions provide robust SSR capabilities, ensuring better SEO and faster initial load times.
Static Site Generation (SSG): The ability to pre-render pages at build time remains consistent, offering improved performance for static content.
API Routes: Both versions continue to support API routes, enabling developers to build full-fledged APIs within their Next.js applications.
Automatic Code Splitting: Both versions ensure that each page only loads what’s necessary, leading to faster page loads and improved performance.
Overview of differences
While Next.js 13 brought numerous enhancements, Next.js 14 takes it a step further with these significant updates:
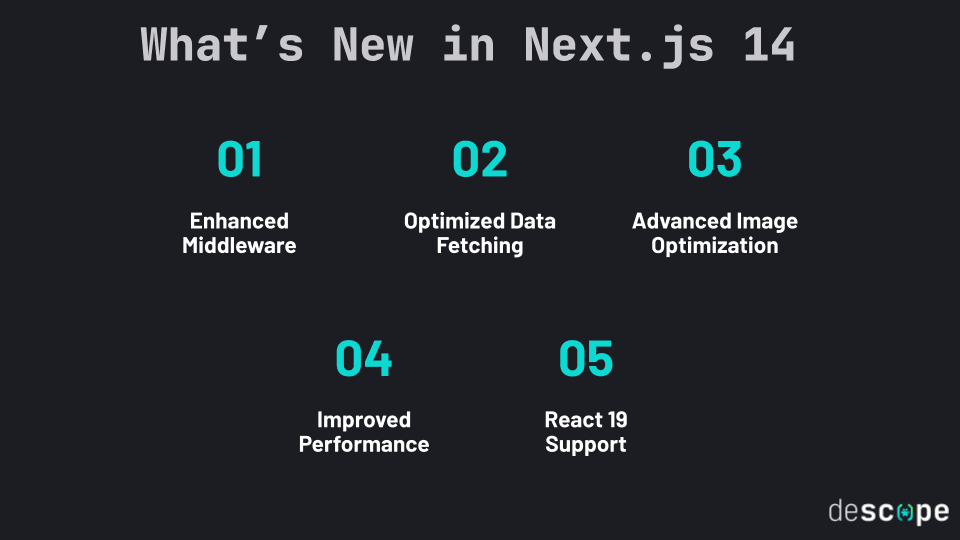
Enhanced Middleware: Next.js 14 includes more robust middleware capabilities, such as conditional request handling depending on URL parameters or headers and advanced request modification.
Optimized Data Fetching: Improvements in data fetching mechanisms, including enhanced support for React Server Components, providing better data-fetching methods that are more efficient and easier to use.
Advanced Image Optimization: A built-in picture optimizer that can considerably reduce the size of your application's image assets, including automatic format detection and adaptive image loading based on device capabilities.
Performance Improvements: Include a new incremental static regeneration (ISR) technique and a speedier development server.
React 19 Support: This includes features like concurrent rendering and the useTransition hook.
Enhanced middleware
Next.js 13
In Next.js 13, the middleware capabilities were evolved, but there was a limit on versatility. The middleware functions were used to intercept requests and responses - which allowed for basic authentication and logging in. It became difficult to handle more complex requests or handle more advanced use cases.
For example, in the code sample below, the middleware function checks for an ‘Authorization’ header and returns ‘Unauthorized’ if it is missing. This works, but is a basic level of request handling in Next.js 13.
//Next.js 13 middleware function example
export function middleware(req){
if(!req.headers.get('Authorization')){
return new Response('Unauthorized', {status: 401});
}
return new Response('OK');
}
Next.js 14
Next.js 14 has the ability to handle more complex requests, with new enhanced middleware abilities. Now, developers can create more sophisticated middleware functions due to conditional request handling, enhanced security, and complex request transformations.
In the code sample below, the middleware function checks for the Authorization
header, and redirects the user to the login page if the header is missing. Additionally, there is a conditional check to return a JSON response if the url
request is an API route.
// Next.js 14 Middleware Example
export function middleware(req) {
const url = new URL(req.url);
// Enhanced request manipulation
if (!req.headers.get('Authorization')) {
url.pathname = '/login';
return Response.redirect(url);
}
// Conditional handling based on URL parameters
if (url.pathname.startsWith('/api')) {
return new Response(JSON.stringify({ message: 'API route accessed' }), { status: 200 });
}
return new Response('OK');
}
Creating a URL object from the request URL allows you to easily perform operations like checking the pathname and modifying it if necessary. With the url
object, you can:
Parse the URL: The URL constructor parses the request URL and provides an object with properties such as protocol, hostname, pathname, search, and hash.
Inspect the URL: You can inspect different parts of the URL to make decisions based on the request. For example, you might want to check the pathname to see if the request is for an API route or a specific page.
Modify the URL: You can modify parts of the URL, such as changing the pathname or adding search parameters. This can be useful for redirecting requests or rewriting URLs.
Optimized data fetching
Next.js 13
In Next.js 13, data fetching was mostly accomplished by methods such as getStaticProps
, getServerSideProps
, and getInitialProps
. These techniques allowed for more flexibility in requesting data throughout the construction process, on each request, or before generating the page on the client side.
// pages/index.js
export async function getServerSideProps() {
// Fetch data from an external API
const res = await fetch('https://api.example.com/data');
const data = await res.json();
// Pass data to the page via props
return { props: { data } };
}
const HomePage = ({ data }) => (
<div>
<h1>Data from API</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
export default HomePage;
In the above example, the getServerSideProps()
fetches the data from the API and passes it to the HomePage as props. Props (short for properties) are a React component that allows you to transmit data from a parent to a child. Props are used to customize and configure child components, allowing them to accept variables from the parent component.
Next.js 14
Next.js 14 introduces improvements in data fetching mechanisms, particularly with enhanced support for React Server Components and new methods that provide more efficient and flexible data-fetching strategies.
// components/DataComponent.server.js
import React from 'react';
export default async function DataComponent() {
// Fetch data from an external API
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return (
<div>
<h1>Data from API</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
// pages/index.js
import dynamic from 'next/dynamic';
// Dynamically import the server component
const DataComponent = dynamic(() => import('../components/DataComponent.server'), {
ssr: true,
});
const HomePage = () => (
<div>
<DataComponent />
</div>
);
export default HomePage;
In the code example above, there are a few key differences that make Next.js 14 a more enhanced framework for data fetching methods.
React Server Components enable server-side rendering of components, resulting in shorter load times and higher performance because only relevant data is delivered to the client.
Additionally, Next.js 14's support for React Server Components provides more flexibility and advanced data-fetching and rendering methodologies, allowing developers to build more dynamic and responsive applications.
The new data fetching methods in Next.js 14 eliminate the need for boilerplate code, making the process easier to understand and manage. Boilerplate code refers to sections of code that are repeated in multiple places with little to no modification.
Advanced image optimization
Next.js 13
Next.js 13 added built-in image optimization capabilities, allowing developers to easily optimize and resize photos without relying on additional plugins or services. This feature has been extended in Next.js 14.
In Next.js 13, the next/image
component is used to handle image optimization. It provides built-in support for resizing, lazy loading, and optimizing images.
// pages/index.js
import Image from 'next/image';
const HomePage = () => (
<div>
<h1>Next.js 13 Image Optimization</h1>
<Image
src="/images/sample.jpg"
alt="Sample Image"
width={500}
height={300}
/>
</div>
);
export default HomePage;
In this code example, the Image component automatically optimizes the image at the specified dimensions (width and height). The optimization includes resizing the image and applying lazy loading.
Next.js 14
Next.js 14 includes support for sophisticated picture formats such as WebP and AVIF, which provide superior compression and reduced file sizes than classic formats like JPEG and PNG. Next.js 14 automatically determines the best format based on the client's browser compatibility, ensuring that users receive the most efficient picture format.
// pages/index.js
import Image from 'next/image';
const HomePage = () => (
<div>
<h1>Next.js 14 Image Optimization</h1>
<Image
src="/images/sample.jpg"
alt="Sample Image"
width={500}
height={300}
// New attributes in Next.js 14 for advanced formats
formats={['image/avif', 'image/webp']}
/>
</div>
);
export default HomePage;
In the above example, the Image component includes a new formats attribute, which specifies the preferred image formats (image/avif and image/webp). Next.js 14 automatically detects the client's browser capabilities and serves the best possible format.
Here are the differences laid out in a table so you can easily compare and understand the updates!
| Next.js 13 | Next.js 14 |
---|---|---|
Advanced Image Formats | Supports basic image optimization with standard formats like JPEG and PNG. | Adds support for advanced image formats like WebP and AVIF, which offer better compression and smaller file sizes. |
Automatic Format Detection | Optimizes images but does not automatically select the optimal format based on the client's browser support. | Automatically detects the client's browser capabilities and serves the most efficient image format (e.g., WebP or AVIF). |
New Attributes for Image Component | Basic attributes for resizing and lazy loading. | Introduces new attributes (like formats) to specify preferred image formats, enhancing the flexibility and efficiency of image optimization. |
Performance improvements
Next.js 13
ISR allows Next.js to generate static pages for your application at build time. This can improve the performance of your application by reducing the number of requests that need to be made to the server. In Next.js 13, ISR was introduced, allowing pages to be statically generated and then updated incrementally after the initial build.
...
export async function getStaticProps({ params }) {
const post = await getPost(params.id);
return {
props: {
post,
},
revalidate: 10, // In seconds
};
}
...
In the example above, getStaticProps generates static pages at build time and revalidates the page every 10 seconds, ensuring that the page is updated with new data.
Next.js 14
Next.js 14 improves the efficiency of the ISR algorithm. The new algorithm reduces the time taken to revalidate pages and includes better handling of updates.
...
export async function getStaticProps({ params }) {
const post = await getPost(params.id);
return {
props: {
post,
},
revalidate: { interval: 10, swr: true }, // Enhanced ISR configuration
};
}
...
The revalidate option has been improved in the above example to incorporate extra options, including swr for stale-while-revalidate support and interval for revalidation time. As a result, ISR in Next.js 14 is now more effective and better equipped to handle changes.
| ISR Configuration | Efficiency and Performance | Development Server Speed |
---|---|---|---|
Next.js 13 | Basic revalidate option with a time interval in seconds. | Introduced ISR, improving performance by generating static pages at build time and revalidating them at specified intervals.
| Standard development server speed. |
Next.js 14 | Enhanced revalidate option that supports more configurations such as interval and swr. | Improved ISR algorithm, reducing revalidation time and handling updates more efficiently, leading to better performance. | Faster development server, providing a more responsive development experience. |
Choosing between Next.js 13 and 14
It can be difficult to choose between Next.js 13 and Next.js 14, but ultimately it comes down to the requirements and objectives of your project. For projects where stability and compatibility are top priorities, Next.js 13 is a solid and dependable option.
However, Next.js 14 is the better option if you want to take advantage of state-of-the-art performance enhancements including expanded middleware capabilities, more effective incremental static regeneration (ISR), and advanced image optimization with WebP and AVIF compatibility. Furthermore, Next.js 14 provides support for the most recent React features, like parallel rendering and the useTransition hook.
In the end, think about what your project needs, how much the new features can help, and whether you're ready to switch to the most recent version. This should help you make the best choice for your application.
Conclusion
As Next.js continues to evolve, I’m sure we’ll get to see many more updates in the upcoming future - so keep an eye out for that! In the meantime, if you would like to know more about the ins-and-outs of Next.js, check out this blog on how to add authentication to a Next.js 13 app using Descope and NextAuth.
Descope is a platform for identity management and authentication for developers that requires little to no code. Feel free to sign up for a ‘Free Forever’ account at this link and start building your next Next.js app (😃). We fully support Next.js and have many sample apps and guides to help you get started with all things auth for your app <3.
Happy coding!