Table of Contents
Basic authentication
This tutorial was written by Ahilya Kulkarni, a software developer and technical writer with a diverse portfolio spanning more than a decade. Connect with them on their LinkedIn to see more of their work!
A solid understanding of authentication is essential for building secure applications. Authentication validates identities before granting access to protected resources, preventing unauthorized access and data breaches. Without proper safeguards in place, APIs, web applications, and services become vulnerable to unauthorized access, data breaches, and security threats. For example, in a cloud-based financial app, weak or missing authentication could allow attackers to exploit predictable user identifiers, automate requests, and extract financial data.
Two commonly used authentication methods are HTTP basic authentication and JWT-based authentication—each with distinct strengths and weaknesses. While the term “basic authentication” may seem straightforward, it can refer to both general authentication principles and a specific method known as “HTTP basic authentication.” This distinction is important as many developers looking for basic authentication may not realize they are referring to a predefined authentication method.
This article explores the key differences between HTTP basic authentication and JWT-based authentication. It also covers the pros and cons of each approach, including when to use them and why JWT-based authentication is generally the preferred choice for most modern applications.
Basic authentication
HTTP authentication provides a standardized framework for securing access to resources through the verification of client credentials. In a general HTTP authentication framework, the client sends a request to a server, the server challenges the client’s request, and the client provides the relevant authentication information. The basic authentication scheme, defined in RFC 7617, is one implementation of this framework.
The following apply in basic authentication:
When a client requests a protected resource, the server responds to an unauthorized request with a
401 Unauthorized
status and aWWW-Authenticate: Basic
header.The client resends the request with an authorization header containing Base64-encoded credentials in the format
username:password
.If the request is valid, the server grants access to the resource with
200 OK status
; otherwise, it returns401 Unauthorized
.
For example, if your username and password are foo
and bar
, the combination foo:bar
is Base64 encoded as Zm9vOmJhcg==
. In this case, the request header would look like this:
Authorization: Basic Zm9vOmJhcg==
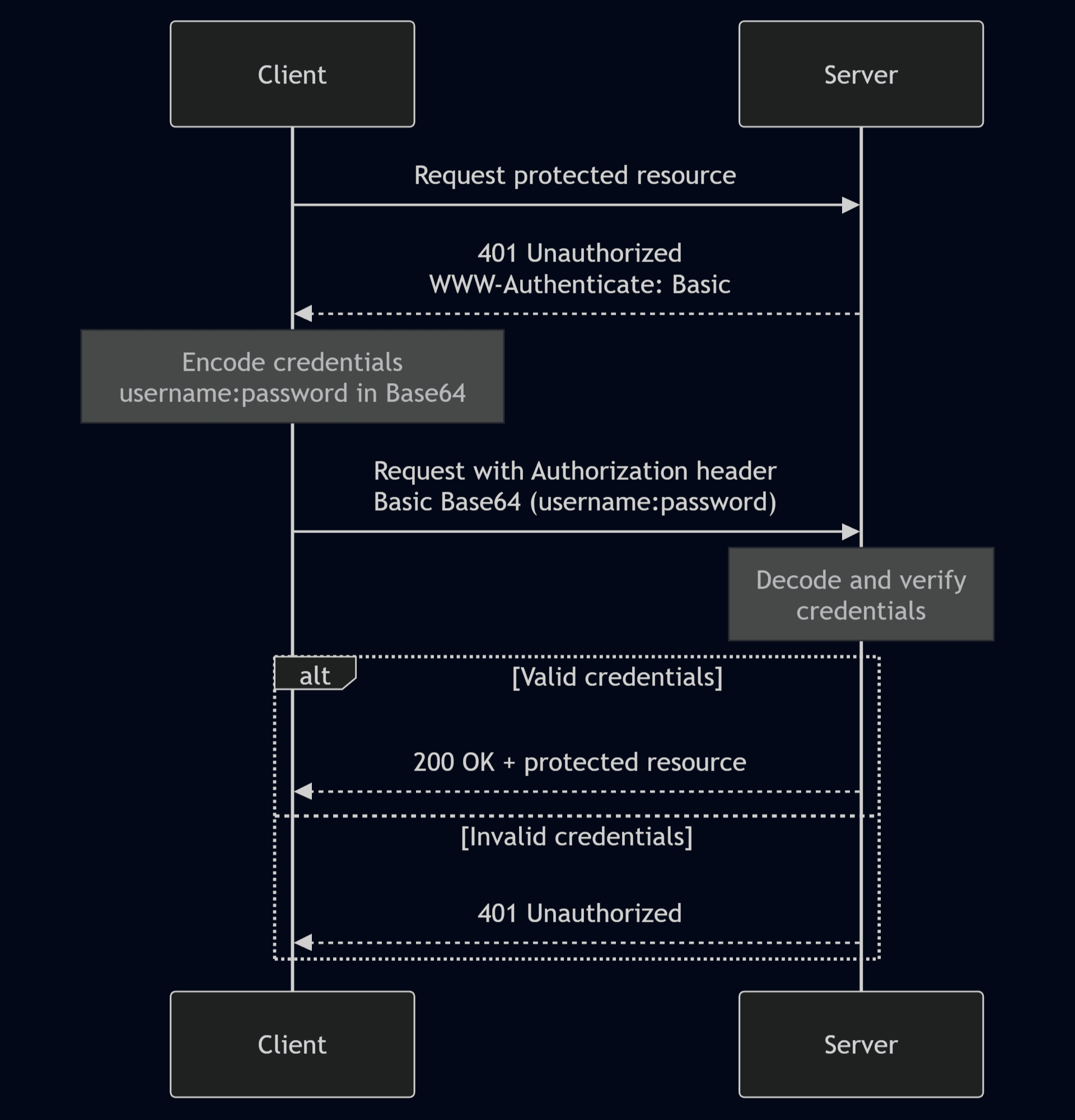
Below is a Node.js example that implements basic authentication:
const express = require('express');
const app = express();
// Basic Auth middleware
function basicAuth(req, res, next) {
// Check for Authorization header
const authHeader = req.headers.authorization;
if (!authHeader || !authHeader.startsWith('Basic ')) {
res.set('WWW-Authenticate', 'Basic realm="Protected Area"');
return res.status(401).send('Authentication required');
}
// Decode credentials
const base64Credentials = authHeader.split(' ')[1];
const credentials = Buffer.from(base64Credentials, 'base64').toString('utf8');
const [username, password] = credentials.split(':');
// Validate credentials
if (username === 'foo' && password === 'bar') {
next();
} else {
res.set('WWW-Authenticate', 'Basic realm="Protected Area"');
return res.status(401).send('Invalid authentication credentials');
}
}
// Protected route
app.get('/api/protected', basicAuth, (req, res) => {
res.json({ message: 'This is protected data' });
});
app.listen(3000, () => console.log('Server running on port 3000'));
Limitations of basic authentication
The following are some limitations and vulnerabilities of basic authentication:
Credentials are transmitted with every request, increasing the risk of exposure.
Base64 encoding offers no real security as it can be easily decoded.
Users must reauthenticate for every request unless the client caches credentials.
If not used over HTTPS, credentials can be intercepted and stolen.
There is no built-in support for additional security layers like MFA or one-time passwords (OTPs).
Basic authentication is mainly used in scenarios where security requirements are minimal or where additional security measures like HTTPS are already in place.
AI tools like Ollama and Silly Tavern often use basic authentication, particularly on self-hosted servers, to restrict access without requiring OAuth or token-based authentication. Developers also use it in staging environments or local development setups to prevent unauthorized access while keeping implementation simple.
JWT-based authentication
A JSON Web Token (JWT) provides a secure and compact way to transmit information between parties in a self-contained JSON object. Each JWT consists of three parts: a header, a payload, and a signature.
Header: The header specifies the token type (JWT) and signing algorithm (eg HS256).
Example:
{
"alg": "HS256",
"typ": "JWT"
}
Payload: The payload contains claims (statements about the user and metadata), which can be of three types:
Registered: Predefined claims such as iss (issuer), exp (expiration time), and sub (subject)
Public: Custom claims that can be shared between parties
Private: Custom claims defined for internal use
Example:
{
"sub": "1234567890",
"name": "John Doe",
"admin": true,
"exp": 1718192000
}
Signature: The signature verifies token integrity. It’s created by encoding the header and payload and signing them with a secret key (HMAC) or private key (RSA).
Example:
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
secret)
A complete JWT consists of three Base64-URL-encoded strings separated by dots, like header.payload.signature
, as shown:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWUsImV4cCI6MTcxODE5MjAwMH0.CpEJCpqBYrqcLVWCctaxxQMayT5FmsEhZBB39zno2Uc
JWT authentication process
The JWT authentication process works as follows:
Token issuance: When a user logs in with their credentials, the server verifies them and generates a JWT containing the user’s identity, roles, and permissions. The token is cryptographically signed using either a secret key or a private key to prevent tampering. Once generated, the token is sent back to the client.
Token storage: The client stores the JWT in one of the following locations:
Local storage (susceptible to XSS attacks, not recommended)
Session storage (cleared when the tab is closed)
Secure HTTP-only cookies (recommended for enhanced security)
More information about token storage can be found here.
Token usage and validation: The client includes the JWT with each request in the HTTP authorization header. The server extracts the JWT, verifies its signature using the stored secret or public key, and checks the token’s expiration and validity. If valid, the request proceeds with the authenticated user’s permissions.
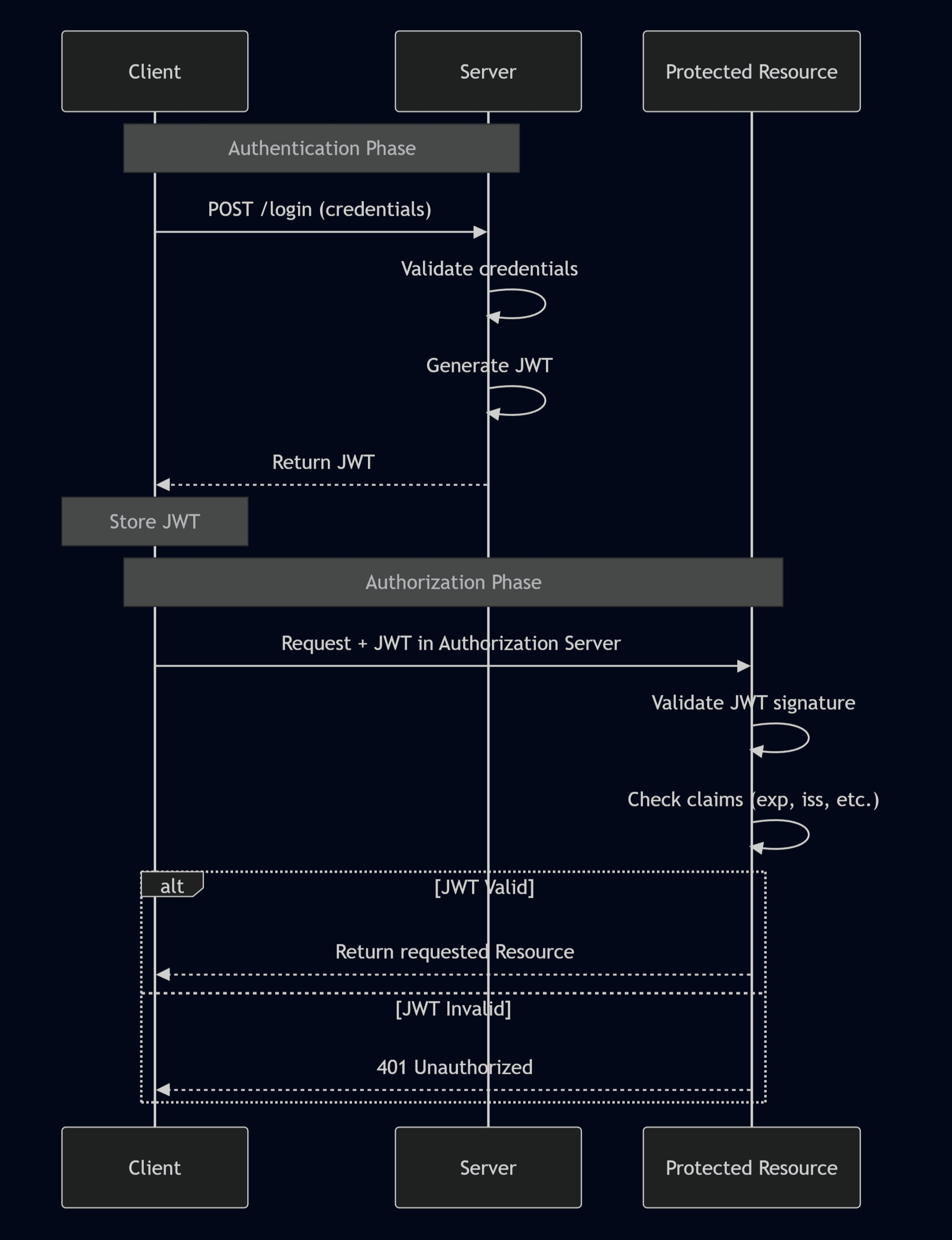
Below is a Node.js example that implements JWT-based authentication:
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
app.use(express.json());
const JWT_SECRET = 'your-secret-key'; // In production, use env variables
// Login endpoint to issue JWT
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Validate credentials (in production, check against database)
if (username === 'foo' && password === 'bar') {
// Create token with expiration
const token = jwt.sign(
{ sub: '1234', username, roles: ['user'] },
JWT_SECRET,
{ expiresIn: '1h' }
);
res.json({ token });
} else {
res.status(401).json({ error: 'Invalid credentials' });
}
});
// JWT authentication middleware
function authenticateJWT(req, res, next) {
const authHeader = req.headers.authorization;
if (!authHeader || !authHeader.startsWith('Bearer ')) {
return res.status(401).json({ error: 'Authentication required' });
}
const token = authHeader.split(' ')[1];
try {
const decoded = jwt.verify(token, JWT_SECRET);
req.user = decoded;
next();
} catch (err) {
return res.status(403).json({ error: 'Invalid or expired token' });
}
}
// Protected route
app.get('/api/protected', authenticateJWT, (req, res) => {
res.json({
message: 'Protected data',
user: req.user
});
});
app.listen(3000, () => console.log('Server running on port 3000'));
Key differences between basic and JWT-based auth
This section explores the differences between the two authentication mechanisms across three aspects: security, scalability, and implementation complexity.
Security considerations
Basic authentication, even though widely used, has several security limitations. Even when used with HTTPS, it exposes credentials (username and password) in every request through Base64 encoding. This encoding is not encryption, and the credentials are only obscured rather than protected.
Basic authentication lacks a standardized logout mechanism. You should either clear their cached credentials, or the server should implement custom session tracking. It does not provide a built-in mechanism for session expiration or token revocation, making it difficult to force logout or limited access after a security breach. Since credentials are static, an attacker who intercepts a request can reuse them unless additional protection mechanisms (such as nonce values) are implemented.
JWT-based authentication provides enhanced security by issuing time-limited tokens. These tokens are always signed cryptographically and contain claims about the user and their permissions. If the contents of a JWT are modified in any way, the signature verification will fail, making the token unusable. This tamper-proofing, especially when implemented with asymmetric keys, allows services to trust the token’s contents for permissions, scopes, and user identity without needing additional verification. Instead of transmitting credentials with every request, a JWT is issued after authentication and used for subsequent requests.
JWTs are typically specific to one service as each service has its own private key for signing tokens. This convention ensures that only the intended service can generate valid tokens and verify their authenticity. That said, applications can also support multiple JWTs by accepting tokens from different issuers, each with its own signing key. For example, a web app might allow users to log in using Google, Facebook, or a custom authentication system, each generating its own JWT. In a microservices setup, different services may issue their own JWTs for internal communication and also accept user tokens from a shared authentication server.
Another example of this approach is seen in integrations between third-party authentication providers like Descope and backend SaaS platforms like Firebase or Supabase. For instance, in this sample application, Descope handles user login on the frontend and issues a session JWT. The app then generates a new JWT signed with Supabase’s secret, embedding the Descope user ID as a claim. Supabase verifies this token and identifies the user, enabling secure authentication across both platforms.
JWTs enable fine-grained permission control using scoped claims to define and restrict user access. These claims can specify exactly what actions a token holder is authorized to perform. This is particularly valuable in microservices architecture, where different services require different levels of access.
Scalability and performance
In basic authentication, servers need to authenticate every request against a user database by verifying the username and password each time. The repeated transmission and verification of credentials can slow down request handling, especially under heavy traffic. This can become a bottleneck in distributed systems as managing verification across multiple servers may need additional infrastructure, such as centralized services and synchronized databases.
While basic authentication can be considered stateless in that the server does not retain session information, JWTs are also stateless and significantly improve performance. JWTs allow servers to verify the validity of the token itself using a cryptographic signature without needing to query a database. Since all necessary information—including user identity, roles, and permissions—is contained within the token, any server in a cluster can validate the token independently. This eliminates the need for session replication or coordination between servers, which simplifies system architecture and reduces operational overhead. It enables easier horizontal scaling as any server can validate tokens independently.
Additionally, because JWTs do not require database lookups for every request, they improve response times and reduce the load on authentication servers. This makes them more suitable for high-performance applications where scalability is crucial.
Implementation complexity
Basic authentication’s simplicity is one of its main advantages. Implementation requires minimal setup as most web servers and frameworks support it natively. The server simply needs to validate credentials against stored values, which makes it straightforward to configure and maintain. Additionally, since it does not require token generation, key management, or complex cryptographic operations, it’s easy to integrate into legacy systems or applications with lower security requirements. However, this simplicity comes at the cost of flexibility and security.
JWT implementation, in contrast, involves careful consideration of several factors:
Tokens must be securely generated, signed, and verified to prevent unauthorized access.
Public and private keys (or shared secrets) must be properly stored and rotated to maintain security.
Expiration policies must be set to balance security and usability, ensuring tokens do not remain valid indefinitely.
Tokens must be stored securely on the client side and transmitted safely to prevent interception.
Despite these complexities, modern authentication libraries and frameworks have significantly reduced the difficulty of implementing JWTs. Many provide built-in JWT support with secure defaults, so it’s easier to integrate them into applications. These libraries also handle common scenarios—such as token refresh, revocation, and role-based access control (RBAC)—so that developers don’t need to manage every aspect manually. When properly implemented, JWT-based authentication is a scalable, flexible, and more secure alternative to basic authentication.
When to use each method
The following table provides a comparison summary of basic and JWT-based authentication to help you choose the right method according to your needs:
Feature | Basic Authentication | JWT-based Authentication |
---|---|---|
Credential Handling | Sent with every request | One-time verification, then token-based |
Security Level | Lower (Base64 encoded) | Higher (cryptographically signed) |
Scalability | Limited | Excellent for distributed systems |
Implementation | Simple | More complex but well supported |
Session Management | No built-in expiration | Configurable expiration |
Logout Mechanism | No standard mechanism | Token invalidation/expiration |
Basic authentication is most appropriate for the following scenarios:
Internal or legacy APIs where advanced authentication is unnecessary
Local development or testing environments before implementing other authentication methods
Applications with low security requirements or those that do not handle sensitive data
Internal tools with limited access and strict firewall policies
Simple API integrations where the client is trusted
Quick prototypes and proofs of concept where speed is important
JWT-based authentication is better suited for the following:
Production applications handling sensitive user data and requiring strong security guarantees
Distributed systems and microservices architecture requiring stateless authentication
Mobile applications that need offline access and secure token storage capabilities
Single-page applications (SPAs) that make frequent API calls (benefitting from reduced server load)
Multitenant systems requiring fine-grained access control through custom claims
Applications implementing single sign-on (SSO) across multiple domains or services
Systems with specific compliance requirements around authentication and authorization
High-traffic applications that need to scale horizontally without shared session storage.
So which one should you choose? If you need a higher level of security with better protection against credential theft and interception, along with scalability, JWT is the best option. If you just need a simple, temporary fix for internal tools, basic authentication might do the job—just don’t use it in production! By following industry best practices, such as those from NIST, OWASP, and major cloud providers like AWS, you can build a stronger and more reliable authentication strategy.
Conclusion
This article explored HTTP basic authentication and JWT-based authentication, highlighting their differences in security, implementation complexity, and scalability. While basic authentication is simple to implement, it poses significant security risks if not used with additional protections, like HTTPS. On the other hand, JWTs offer a more flexible and scalable approach, especially for distributed systems, but require careful token management to avoid vulnerabilities.
By considering factors such as credential exposure, encryption, token storage, and performance, you can make informed decisions about which authentication method best suits your application’s needs.
To keep up to date with more developer deep-dives from the world of identity and authentication, subscribe to our blog or follow us on LinkedIn, X, and Bluesky.